So I have stop loss 6 and take profit 36, but my code only gives me equal stop loss and take profit, so that I can not make any profit. Here is what the results look like. What is wrong?
So the settings and the results do not correlate. Why can I not set stop loss and take profit so that it actually works?
here is my code
package com.dukascopy.visualforex.visualjforex;
import java.util.*; import com.dukascopy.api.*; import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.concurrent.CopyOnWriteArrayList; import java.lang.reflect.*; import java.math.BigDecimal;
/* * Created by VisualJForex Generator, version 3.0.9 * Date: 25.12.2021 07:41 */ public class untitledStrategy1 implements IStrategy {
private CopyOnWriteArrayList<TradeEventAction> tradeEventActions = new CopyOnWriteArrayList<TradeEventAction>(); private static final String DATE_FORMAT_NOW = "yyyyMMdd_HHmmss"; private IEngine engine; private IConsole console; private IHistory history; private IContext context; private IIndicators indicators; private IUserInterface userInterface;
@Configurable("defaultTakeProfit:") public int defaultTakeProfit = 36; @Configurable("defaultTradeAmount:") public double defaultTradeAmount = 0.06; @Configurable("defaultStopLoss:") public int defaultStopLoss = 6; @Configurable("defaultInstrument:") public Instrument defaultInstrument = Instrument.EURUSD; @Configurable("defaultPeriod:") public Period defaultPeriod = Period.ONE_MIN; @Configurable("defaultSlippage:") public int defaultSlippage = 3;
private Candle LastBidCandle = null ; private String AccountId = ""; private List<IOrder> PendingPositions = null ; private double Equity; private List<IOrder> OpenPositions = null ; private Tick LastTick = null ; private String AccountCurrency = ""; private double UseofLeverage; private IMessage LastTradeEvent = null ; private int OverWeekendEndLeverage; private boolean GlobalAccount; private Candle LastAskCandle = null ; private int MarginCutLevel; private List<IOrder> AllPositions = null ; private double Leverage;
public void onStart(IContext context) throws JFException { this.engine = context.getEngine(); this.console = context.getConsole(); this.history = context.getHistory(); this.context = context; this.indicators = context.getIndicators(); this.userInterface = context.getUserInterface();
subscriptionInstrumentCheck(defaultInstrument);
ITick lastITick = context.getHistory().getLastTick(defaultInstrument); LastTick = new Tick(lastITick, defaultInstrument);
IBar bidBar = context.getHistory().getBar(defaultInstrument, defaultPeriod, OfferSide.BID, 1); IBar askBar = context.getHistory().getBar(defaultInstrument, defaultPeriod, OfferSide.ASK, 1); LastAskCandle = new Candle(askBar, defaultPeriod, defaultInstrument, OfferSide.ASK); LastBidCandle = new Candle(bidBar, defaultPeriod, defaultInstrument, OfferSide.BID);
subscriptionInstrumentCheck(Instrument.fromString("EUR/USD"));
}
public void onAccount(IAccount account) throws JFException { AccountCurrency = account.getCurrency().toString(); Leverage = account.getLeverage(); AccountId= account.getAccountId(); Equity = account.getEquity(); UseofLeverage = account.getUseOfLeverage(); OverWeekendEndLeverage = account.getOverWeekEndLeverage(); MarginCutLevel = account.getMarginCutLevel(); GlobalAccount = account.isGlobal(); }
private void updateVariables(Instrument instrument) { try { AllPositions = engine.getOrders(); List<IOrder> listMarket = new ArrayList<IOrder>(); for (IOrder order: AllPositions) { if (order.getState().equals(IOrder.State.FILLED)){ listMarket.add(order); } } List<IOrder> listPending = new ArrayList<IOrder>(); for (IOrder order: AllPositions) { if (order.getState().equals(IOrder.State.OPENED)){ listPending.add(order); } } OpenPositions = listMarket; PendingPositions = listPending; } catch(JFException e) { e.printStackTrace(); } }
public void onMessage(IMessage message) throws JFException { if (message.getOrder() != null) { updateVariables(message.getOrder().getInstrument()); LastTradeEvent = message; for (TradeEventAction event : tradeEventActions) { IOrder order = message.getOrder(); if (order != null && event != null && message.getType().equals(event.getMessageType())&& order.getLabel().equals(event.getPositionLabel())) { Method method; try { method = this.getClass().getDeclaredMethod(event.getNextBlockId(), Integer.class); method.invoke(this, new Integer[] {event.getFlowId()}); } catch (SecurityException e) { e.printStackTrace(); } catch (NoSuchMethodException e) { e.printStackTrace(); } catch (IllegalArgumentException e) { e.printStackTrace(); } catch (IllegalAccessException e) { e.printStackTrace(); } catch (InvocationTargetException e) { e.printStackTrace(); } tradeEventActions.remove(event); } } } }
public void onStop() throws JFException { }
public void onTick(Instrument instrument, ITick tick) throws JFException { LastTick = new Tick(tick, instrument); updateVariables(instrument);
}
public void onBar(Instrument instrument, Period period, IBar askBar, IBar bidBar) throws JFException { LastAskCandle = new Candle(askBar, period, instrument, OfferSide.ASK); LastBidCandle = new Candle(bidBar, period, instrument, OfferSide.BID); updateVariables(instrument); OpenatMarket_block_10(1);
}
public void subscriptionInstrumentCheck(Instrument instrument) { try { Set<Instrument> instruments = new HashSet<Instrument>(); instruments.add(instrument); context.setSubscribedInstruments(instruments, true); } catch (Exception e) { e.printStackTrace(); } }
public double round(double price, Instrument instrument) { BigDecimal big = new BigDecimal("" + price); big = big.setScale(instrument.getPipScale() + 1, BigDecimal.ROUND_HALF_UP); return big.doubleValue(); }
public ITick getLastTick(Instrument instrument) { try { return (context.getHistory().getTick(instrument, 0)); } catch (JFException e) { e.printStackTrace(); } return null; }
private void OpenatMarket_block_10(Integer flow) { Instrument argument_1 = defaultInstrument; double argument_2 = defaultTradeAmount; int argument_3 = defaultSlippage; int argument_4 = defaultStopLoss; int argument_5 = defaultTakeProfit; String argument_6 = ""; ITick tick = getLastTick(argument_1);
IEngine.OrderCommand command = IEngine.OrderCommand.BUY;
double stopLoss = tick.getBid() - argument_1.getPipValue() * argument_4; double takeProfit = round(tick.getBid() + argument_1.getPipValue() * argument_5, argument_1); try { String label = getLabel(); IOrder order = context.getEngine().submitOrder(label, argument_1, command, argument_2, 0, argument_3, stopLoss, takeProfit, 0, argument_6); OpenatMarket_block_11(flow); } catch (JFException e) { e.printStackTrace(); } }
private void OpenatMarket_block_11(Integer flow) { Instrument argument_1 = defaultInstrument; double argument_2 = defaultTradeAmount; int argument_3 = defaultSlippage; int argument_4 = 25; int argument_5 = defaultTakeProfit; String argument_6 = ""; ITick tick = getLastTick(argument_1);
IEngine.OrderCommand command = IEngine.OrderCommand.SELL;
double stopLoss = tick.getAsk() + argument_1.getPipValue() * argument_4; double takeProfit = round(tick.getAsk() - argument_1.getPipValue() * argument_5, argument_1); try { String label = getLabel(); IOrder order = context.getEngine().submitOrder(label, argument_1, command, argument_2, 0, argument_3, stopLoss, takeProfit, 0, argument_6); } catch (JFException e) { e.printStackTrace(); } }
class Candle {
IBar bar; Period period; Instrument instrument; OfferSide offerSide;
public Candle(IBar bar, Period period, Instrument instrument, OfferSide offerSide) { this.bar = bar; this.period = period; this.instrument = instrument; this.offerSide = offerSide; }
public Period getPeriod() { return period; }
public void setPeriod(Period period) { this.period = period; }
public Instrument getInstrument() { return instrument; }
public void setInstrument(Instrument instrument) { this.instrument = instrument; }
public OfferSide getOfferSide() { return offerSide; }
public void setOfferSide(OfferSide offerSide) { this.offerSide = offerSide; }
public IBar getBar() { return bar; }
public void setBar(IBar bar) { this.bar = bar; }
public long getTime() { return bar.getTime(); }
public double getOpen() { return bar.getOpen(); }
public double getClose() { return bar.getClose(); }
public double getLow() { return bar.getLow(); }
public double getHigh() { return bar.getHigh(); }
public double getVolume() { return bar.getVolume(); } } class Tick {
private ITick tick; private Instrument instrument;
public Tick(ITick tick, Instrument instrument){ this.instrument = instrument; this.tick = tick; }
public Instrument getInstrument(){ return instrument; }
public double getAsk(){ return tick.getAsk(); }
public double getBid(){ return tick.getBid(); }
public double getAskVolume(){ return tick.getAskVolume(); }
public double getBidVolume(){ return tick.getBidVolume(); }
public long getTime(){ return tick.getTime(); }
public ITick getTick(){ return tick; } }
protected String getLabel() { String label; label = "IVF" + getCurrentTime(LastTick.getTime()) + generateRandom(10000) + generateRandom(10000); return label; }
private String getCurrentTime(long time) { SimpleDateFormat sdf = new SimpleDateFormat(DATE_FORMAT_NOW); return sdf.format(time); }
private static String generateRandom(int n) { int randomNumber = (int) (Math.random() * n); String answer = "" + randomNumber; if (answer.length() > 3) { answer = answer.substring(0, 4); } return answer; }
class TradeEventAction { private IMessage.Type messageType; private String nextBlockId = ""; private String positionLabel = ""; private int flowId = 0;
public IMessage.Type getMessageType() { return messageType; }
public void setMessageType(IMessage.Type messageType) { this.messageType = messageType; }
public String getNextBlockId() { return nextBlockId; }
public void setNextBlockId(String nextBlockId) { this.nextBlockId = nextBlockId; } public String getPositionLabel() { return positionLabel; }
public void setPositionLabel(String positionLabel) { this.positionLabel = positionLabel; } public int getFlowId() { return flowId; } public void setFlowId(int flowId) { this.flowId = flowId; } } }
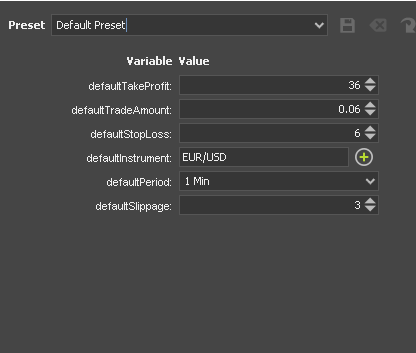
Attachments: |
File comment: and here are the results...
error22.PNG [16.86 KiB]
Downloaded 263 times
|
File comment: Here is my setup
error.PNG [5.99 KiB]
Downloaded 263 times
|
|