|
Jforex programming education |
Peggy37
|
Post subject: Jforex programming education |
Post rating: 0
|
Posted: Sun 28 Feb, 2016, 20:06
|
|
User rating: 0
Joined: Fri 04 Sep, 2015, 19:44 Posts: 34 Location: Slovakia, KE
|
Hi, i want to make me simple strategy (i have many free time) and i need questions about:
0. Where / into what sections to write a code 1. Getting charts , asks, bids from 1 pair 2. Deciding of size and amount of candles 3. Buy/ sell conditions - min. 1 hour trade, max 4 hours 4. Filtering weekends 5. Preventing from opening more than 1 position / time 6. If i need additional classes / functions / declarations... 7. The more automated as it can be - i don' t need many @configurable variables. Are they banned in strategy contest ? 8. Reading from calendar and deciding on values when to buy / sell 9. What is OHLC index ? and do i need this?
I'm looking for some "manual" , i didn't find anything complex on internet to suit to my needs.
Thanks.
|
|
|
|
 |
al_dcdemo
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Tue 01 Mar, 2016, 10:25
|
|
User rating: 3
Joined: Wed 18 Sep, 2013, 23:40 Posts: 13 Location: SloveniaSlovenia
|
package jforex;
import com.dukascopy.api.IAccount; import com.dukascopy.api.IBar; import com.dukascopy.api.IConsole; import com.dukascopy.api.IContext; import com.dukascopy.api.IEngine; import com.dukascopy.api.IMessage; import com.dukascopy.api.IStrategy; import com.dukascopy.api.ITick; import com.dukascopy.api.Instrument; import com.dukascopy.api.JFException; import com.dukascopy.api.Period;
import java.util.Calendar; import java.util.Set; import java.util.TimeZone;
public class Examples implements IStrategy {
private IContext context; // Declare a member variable of type IContext private IConsole console; // Declare a member variable of type IConsole private IEngine engine; // Declare a member variable of type IEngine
@Override public void onStart(IContext context) throws JFException { this.context = context; // Save IContext to a member variable for easy access this.console = context.getConsole(); // Save IConsole to a member variable for easy access this.engine = context.getEngine(); // Save IEngine to a member variable for easy access
// You have to subscribe to all instruments that you intend to use in order to run strategy on a remote server (also applies to Strategy Contest strategies). Set subscribedInstruments = context.getSubscribedInstruments(); // Obtain an empty set. A "set" is an unordered collection that contains zero or more unique elements. subscribedInstruments.add(Instrument.EURUSD); // Add EURUSD instrument to the set subscribedInstruments.add(Instrument.USDJPY); // Add USDJPY instrument to the set context.setSubscribedInstruments(subscribedInstruments); // Pass the updated set back to the context }
@Override public void onStop() throws JFException { }
@Override public void onAccount(IAccount account) throws JFException { }
@Override public void onMessage(IMessage message) throws JFException { }
@Override public void onBar(Instrument instrument, Period period, IBar askBar, IBar bidBar) throws JFException { if (isWeekDay(askBar.getTime())) { // Filtering weekends (4.) if (instrument == Instrument.EURUSD) { // Filter by one instrument (1.) if (period == Period.ONE_HOUR) { // Filter by period (3.) double ask = askBar.getOpen(); // Get ask (1.) double bid = bidBar.getOpen(); // Get bid (1.) double askBarOpen = askBar.getOpen(); // Get ask bar open (2.) double askBarHigh = askBar.getHigh(); // Get ask bar high (2.) double askBarLow = askBar.getLow(); // Get ask bar low (2.) double askBarClose = askBar.getClose(); // Get ask bar close (2.) double askBarVolume = askBar.getVolume(); // Get ask bar volume (2.) double bidBarOpen = bidBar.getOpen(); // Get bid bar open (2.) double bidBarHigh = bidBar.getHigh(); // Get bid bar high (2.) double bidBarLow = bidBar.getLow(); // Get bid bar low (2.) double bidBarClose = bidBar.getClose(); // Get bid bar close (2.) double bidBarVolume = bidBar.getVolume(); // Get bid bar volume (2.) if (engine.getOrders().size() == 0) { // Prevent from opening more than 1 position (5.) // Open new position here } } } } }
@Override public void onTick(Instrument instrument, ITick tick) throws JFException { if (isWeekDay(tick.getTime())) { // Filtering weekends (4.) if (instrument == Instrument.EURUSD || instrument == Instrument.USDJPY) { // Filter by two instruments (1.) double ask = tick.getAsk(); // Get ask (1.) double bid = tick.getBid(); // Get bid (1.) if (engine.getOrders().size() == 0) { // Prevent from opening more than 1 position (5.) // Open new position here } } } }
// Returns true if specified time belongs to a week day, false otherwise private boolean isWeekDay(long time) { Calendar calendar = Calendar.getInstance(TimeZone.getTimeZone("GMT")); // Obtain Calendar object with timezone GMT calendar.setTimeInMillis(time); // Set time
int dayOfWeek = calendar.get(Calendar.DAY_OF_WEEK); // Get day of the week return (dayOfWeek >= Calendar.MONDAY) && (dayOfWeek <= Calendar.FRIDAY); // Returns true if dayOfWeek is between MONDAY and FRIDAY (inclusive), false otherwise } }
Peggy37 wrote: 0. Where / into what sections to write a code All sections (onStart, onStop, onAccount, onMessage, onBar and onTick methods) have their own purpose but you'd mostly use onStart for initialization and onBar or onTick for trading code. Peggy37 wrote: 1. Getting charts, asks, bids from 1 pair See onBar and onTick methods. Peggy37 wrote: 2. Deciding of size and amount of candles See onBar method. Peggy37 wrote: 3. Buy/ sell conditions - min. 1 hour trade, max 4 hours See onBar method. Peggy37 wrote: 4. Filtering weekends See onBar, onTick and isWeekDay methods. Peggy37 wrote: 5. Preventing from opening more than 1 position / time See onBar and onTick methods. Peggy37 wrote: 6. If i need additional classes / functions / declarations... You can use all Java language features in JForex strategy code, though there are some limitations when it comes to remote strategies: https://www.dukascopy.com/wiki/#Remote_RunPeggy37 wrote: 7. The more automated as it can be - i don' t need many @configurable variables. Are they banned in strategy contest ? @Configurable variables (a.k.a. parameters) come in handy, so that you don't need to recompile your strategy each time you change something (SL, TP, trade amount, ...). They're not banned in the strategy contest. Peggy37 wrote: 8. Reading from calendar and deciding on values when to buy / sell I haven't used it in my code yet but I'd look here if I'd decide to use it: https://www.dukascopy.com/wiki/#Trade_on_newsPeggy37 wrote: 9. What is OHLC index ? and do i need this? OHLC index is a chart tool which allows you to check each candle details: date, time, open, high, low, close and volume. You don't have to have it on your charts if you don't need it. Peggy37 wrote: I'm looking for some "manual" , I didn't find anything complex on internet to suit to my needs. Open wiki: https://www.dukascopy.com/wiki/Browse "Strategy API" folder and its subfolders, you have all sorts of examples (simple and complex) there, pertaining to all aspects of JForex programming. NOTE: The above code is just for illustration purposes and is not how a complete and working strategy would look like. Most of the times you'd use just onBar or onTick method but rarely both of them at the same time.
|
|
|
|
 |
Peggy37
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Tue 01 Mar, 2016, 17:22
|
|
User rating: 0
Joined: Fri 04 Sep, 2015, 19:44 Posts: 34 Location: Slovakia, KE
|
Thank you! I understand it more.
|
|
|
|
 |
Peggy37
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Tue 01 Mar, 2016, 22:40
|
|
User rating: 0
Joined: Fri 04 Sep, 2015, 19:44 Posts: 34 Location: Slovakia, KE
|
In example BarsAndTicks - from manual get this error message: Mandatory parameter "Offer Side Value" is not set. 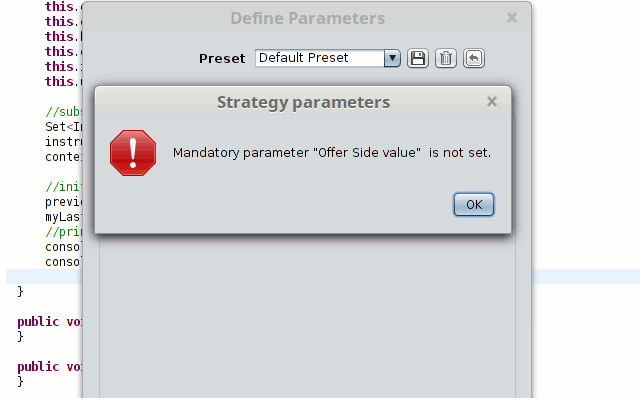
Attachments: |
error.gif [18.05 KiB]
Downloaded 842 times
|
DISCLAIMER: Dukascopy Bank SA's waiver of responsability - Documents, data or information available on
this webpage may be posted by third parties without Dukascopy Bank SA being obliged to make any control
on their content. Anyone accessing this webpage and downloading or otherwise making use of any document,
data or information found on this webpage shall do it on his/her own risks without any recourse against
Dukascopy Bank SA in relation thereto or for any consequences arising to him/her or any third party from
the use and/or reliance on any document, data or information found on this webpage.
|
|
|
|
|
 |
Peggy37
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Tue 01 Mar, 2016, 23:30
|
|
User rating: 0
Joined: Fri 04 Sep, 2015, 19:44 Posts: 34 Location: Slovakia, KE
|
Is it possible the strategy to decide on 3 green same bars in a row, and then buy, not the 1 bar as in manual? Where i put for(bar=0; bar=3; bar++) or in java ?
|
|
|
|
 |
Peggy37
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Wed 02 Mar, 2016, 18:04
|
|
User rating: 0
Joined: Fri 04 Sep, 2015, 19:44 Posts: 34 Location: Slovakia, KE
|
I can't remove this error message,please help !
|
|
|
|
 |
Peggy37
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Thu 03 Mar, 2016, 17:29
|
|
User rating: 0
Joined: Fri 04 Sep, 2015, 19:44 Posts: 34 Location: Slovakia, KE
|
I corrected it: 1. public OfferSide myOfferSide = OfferSide.BID; 2. Set subscribedInstruments = context.getSubscribedInstruments(); subscribedInstruments.add(myInstrument); // USD/CAD context.setSubscribedInstruments(subscribedInstruments); is it good ? What does it mean ? : (1.), (2.), (5.) ? And @Override ?
|
|
|
|
 |
al_dcdemo
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Sat 05 Mar, 2016, 01:59
|
|
User rating: 3
Joined: Wed 18 Sep, 2013, 23:40 Posts: 13 Location: SloveniaSlovenia
|
Peggy37 wrote: Thank you! I understand it more. I'm glad to hear that! Peggy37 wrote: In example BarsAndTicks - from manual get this error message:
Mandatory parameter "Offer Side Value" is not set. Peggy37 wrote: I can't remove this error message,please help ! Peggy37 wrote: I corrected it:
public OfferSide myOfferSide = OfferSide.BID; You solved it well. Another way would be to just manually select the offer side in the "Define Parameters" window. Peggy37 wrote: Is it possible the strategy to decide on 3 green same bars in a row, and then buy, not the 1 bar as in manual? Where i put for(bar=0; bar=3; bar++) or in java ? boolean threeGreenBars = true; // Declare variable threeGreenBars and set it to true
for (int i = 1; i <= 3; i++) { previousBar = history.getBar(myInstrument, myPeriod, myOfferSide, i); // Get i-th previous bar if (previousBar.getClose() <= previousBar.getOpen()) { // Check if bar's close is lower than or equal to bar's open. In other words, we look for bars that are not green (but either red or flat). threeGreenBars = false; // Set the variable to false break; // We found a bar which is not green, so we break the loop here } }
if (threeGreenBars) { // If the variable remains true, that means all tested bars were green }
Peggy37 wrote: is it good ? The code looks good. Peggy37 wrote: What does it mean ? : (1.), (2.), (5.) ? In (1.) you set the default value of variable myOfferSide to OfferSide.BID. In (2.) you just added the comment // USD/CAD I don't see any (5.) Peggy37 wrote: And @Override ? @Override is an annotation that tells the compiler that the annotated method overrides a method in a superclass or implements a method of an interface (as in our case). BTW: There are many free online Java tutorials but if you want to get seriously into Java programming the following book is a great starting point: https://mindview.net/Books/TIJ4
|
|
|
|
 |
Peggy37
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Sat 05 Mar, 2016, 22:06
|
|
User rating: 0
Joined: Fri 04 Sep, 2015, 19:44 Posts: 34 Location: Slovakia, KE
|
Thank you for super answers. (We didn't have java, only c/c++).
|
|
|
|
 |
ivan_taranov
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Sat 12 Mar, 2016, 14:23
|
|
User rating: 0
Joined: Mon 07 Jan, 2013, 23:30 Posts: 19 Location: Ukraine, Odessa
|
|
|
|
 |
Peggy37
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Fri 25 Mar, 2016, 13:06
|
|
User rating: 0
Joined: Fri 04 Sep, 2015, 19:44 Posts: 34 Location: Slovakia, KE
|
Is this correct? OnStart() = subscribe , draw chart, OnTick() = getting asks/bids (matket deph), getting spread Why do i have OnCandle()? Isn't it better to execute orders based on completed candle() than on bar()? How do a steategy knows if / that a candle is completed? Where to add close orders function based on : if TP / else if SL / else if TP is not reached more than 2 hours from execution? Therés no main() function and i can't get used to it. That manual sucks.! ! When i was trying some example and there wasn't close order "handler" and i had to close it down from mobile phone.  Thanks , if someone replies.
|
|
|
|
 |
ivan_taranov
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Fri 25 Mar, 2016, 18:07
|
|
User rating: 0
Joined: Mon 07 Jan, 2013, 23:30 Posts: 19 Location: Ukraine, Odessa
|
Peggy37 wrote: Is this correct? OnStart() = subscribe , draw chart, OnTick() = getting asks/bids (matket deph), getting spread Why do i have OnCandle()? Isn't it better to execute orders based on completed candle() than on bar()? How do a steategy knows if / that a candle is completed? Where to add close orders function based on : if TP / else if SL / else if TP is not reached more than 2 hours from execution? Therés no main() function and i can't get used to it. That manual sucks.! ! When i was trying some example and there wasn't close order "handler" and i had to close it down from mobile phone.  Thanks , if someone replies. ***unlike of C, functions in JAVA are called - methods*** 1. I don't have onCandle() method, when create a new Strategy template. Only onBar(). 2. The JForex terminal calls a onBar() method, every time when a new bar completed. It's written in the manual here: https://www.dukascopy.com/wiki/#Strategy_API . To understand a logic of any method or class, I recommend to use a tracing. Add a line with console out ( https://www.dukascopy.com/wiki/#IConsole ). Force your strategy to display a content of variables/objects/etc on every iteration. 3. You can add a "close order" inside any method. Doesn't matter is it onBar() or onTick() or etc. How to work without main() function will be more clear, when you will finish a couple of levels in javarush=)). To close an order I use time. To track for this the java class Calendar is good. You will learn it after 4-th or 3-rd level in javarush. 4. The manual is very good) When I began to learn JForex API, I also knew only C++ and it's was unbearable to understand a java syntax, without java knowledge. It will be more clear, when you will finish a couple of levels in javarush))) Believe me) How to close an order is explained here: https://www.dukascopy.com/wiki/#Close_Orders . Good luck!)
|
|
|
|
 |
Peggy37
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Fri 25 Mar, 2016, 20:18
|
|
User rating: 0
Joined: Fri 04 Sep, 2015, 19:44 Posts: 34 Location: Slovakia, KE
|
Thanks, i don't know which javarush level I am, but i make keyboard input tasks.
The API manual is not good at all, because there is wrong "Set " command structure and they just head into indicators after few pages and the methods are not properly explained. How many commands are yet wrong? And when i create new indicator, there's nothing about it - only EXAMPID (or something ) and no default info about it. . I must have at least 1 indicator, because i found, that one of them works very well as "trendless filter".
|
|
|
|
 |
Peggy37
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Thu 31 Mar, 2016, 22:28
|
|
User rating: 0
Joined: Fri 04 Sep, 2015, 19:44 Posts: 34 Location: Slovakia, KE
|
Why open positions onTick + onBar ? Isn't it in "conflict" ? The more i read it, the more I don't understand it. Does that mean that onTick is like "scalping" mode and on "onBar" is "normal" mode? Where exactly do i add ENGINE.SUBMITORDER coomands in this ? (1 for sell(3red) 1 for buy(3green)) boolean threeGreenBars = true; // Declare variable threeGreenBars and set it to true for (int i = 1; i <= 3; i++) { previousBar = history.getBar(myInstrument, myPeriod, myOfferSide, i); // Get i-th previous bar if (previousBar.getClose() <= previousBar.getOpen()) { // Check if bar's close is lower than or equal to bar's open. In other words, we look for bars that are not green (but either red or flat). threeGreenBars = false; // Set the variable to false break; // We found a bar which is not green, so we break the loop here } } if (threeGreenBars) { // If the variable remains true, that means all tested bars were green }
|
|
|
|
 |
Peggy37
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Thu 16 Jun, 2016, 20:29
|
|
User rating: 0
Joined: Fri 04 Sep, 2015, 19:44 Posts: 34 Location: Slovakia, KE
|
1. How to do the strategy to work with candles instead of bars ? Will i get the same results with on bars onBar() ? 2. How to "connect "main function with (selected) indicator ? The indicator only Redraws the chart, there are no parameters, does nothing more. 3. Why the manual is not writtten in "human language" ?
Thanks.
|
|
|
|
 |
tcsabina
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Mon 27 Jun, 2016, 21:21
|
|
User rating: 164
Joined: Mon 08 Oct, 2012, 10:35 Posts: 676 Location: NetherlandsNetherlands
|
|
|
|
 |
Peggy37
|
Post subject: Re: Jforex programming education |
Post rating: 0
|
Posted: Sun 10 Jul, 2016, 09:16
|
|
User rating: 0
Joined: Fri 04 Sep, 2015, 19:44 Posts: 34 Location: Slovakia, KE
|
It´ s not complete enough for my strategy type, but thanks.
How to prevent asymetri? How to prevent strategy overloading?
How (and where) to test for take profit and SL reach?
Thanks.
|
|
|
|
 |
|
Pages: [
1
]
|
|
|
|
|